Enrise Tech - Your coding community ๐๐Youtube Hono Series: Episode 4 Enhance the Tasks API with User Association
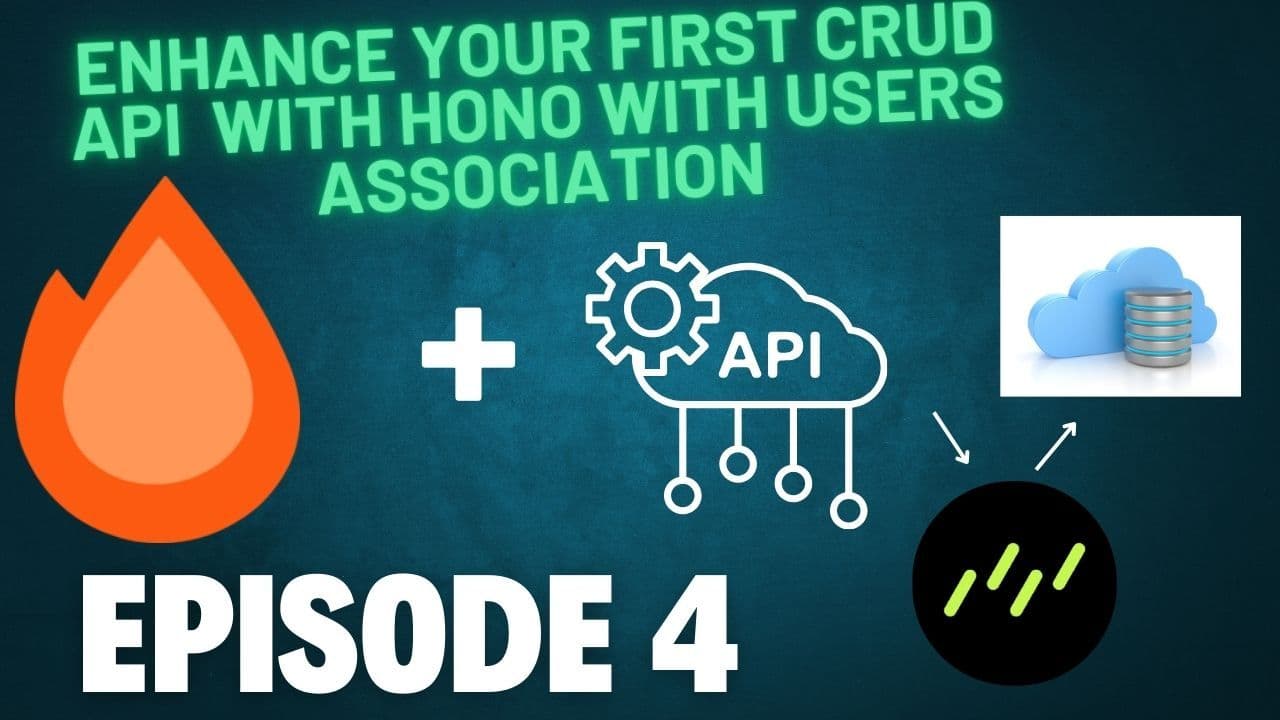
Episode 4 - Enhancing Our Tasks API with User Association ๐
In Episode 4 of our API series, we dive deeper into making our application smarter and more functional by associating tasks with users. This episode is perfect for anyone who wants to add relational functionality to their API while keeping it simple and efficient. Hereโs the breakdown of what we covered:
Updating the Database Schema
Weโve upgraded our database schema to include a users
table and established a foreign key relationship with the tasks
table. Here's the updated schema:
typescriptCopy codeimport { sqliteTable, text, integer, foreignKey } from 'drizzle-orm/sqlite-core';
// Define the Users table
export const users = sqliteTable('users', {
id: integer('id').primaryKey({ autoIncrement: true }),
username: text('username').notNull(),
email: text('email').notNull().unique(),
password: text('password').notNull(),
});
// Define the Tasks table with a foreign key to Usersexport const tasks = sqliteTable('tasks', {
id: integer('id').primaryKey({ autoIncrement: true }),
title: text('title').notNull(),
description: text('description'),
status: text('status').notNull().default('pending'),
userId: integer('user_id')
.references(() => users.id) // Foreign key references `users.id`
.notNull(),
});
We also enabled foreign key constraints in SQLite by adding:
typescriptCopy codesqlite.exec('PRAGMA foreign_keys = ON;'); // Enable foreign key constraints
Database Commands
After updating the schema, we ran the following commands to apply and interact with the database:
- Generate and Migrate Tables:
bashCopy codenpm run db:generate
npm run db:migrate
- Explore with Drizzle Studio:
Launch the studio for direct interaction with your database:
bashCopy codenpm run studio
API Updates for CRUD Operations
To integrate the new users
table into our API, we updated our endpoints for both tasks
and users
. Hereโs a quick summary of the key features:
User Management Endpoints
- Get All Users:
/users
- Get User by ID:
/users/:id
- Create User:
/users
- Update User:
/users/:id
- Delete User:
/users/:id
Enhanced Tasks Endpoints
- Get All Tasks (Optional User Filter):
/tasks
- Get Tasks by User ID:
/tasks/:userId
- Create Task for User:
/tasks
(RequiresuserId
in query params) - Update and Delete Tasks:
/tasks/:id
These additions ensure that every task is associated with a user, making the API scalable and ready for multi-user applications.
Watch the Tutorial
Iโve gone through everything in detail in the video, with a step-by-step explanation of the schema updates, database interactions, and API improvements. Watch it here:
๐ Episode 4: Enhance the Tasks API with User Association
Explore the Codebase
Want to get hands-on? Check out the full code on GitHub:
๐ GitHub Repo: Episode4-UserAssociationWithTasks
Join the Discussion
What challenges have you faced while adding user associations in your APIs? How do you structure your database for similar features? Let me know in the comments below or on Reddit. Letโs build and learn together!
๐ Start Building Your Next App with Confidence! ๐