Enrise Tech - Your coding community 😉🚀YouTube Hono Series: First HONO CRUD API
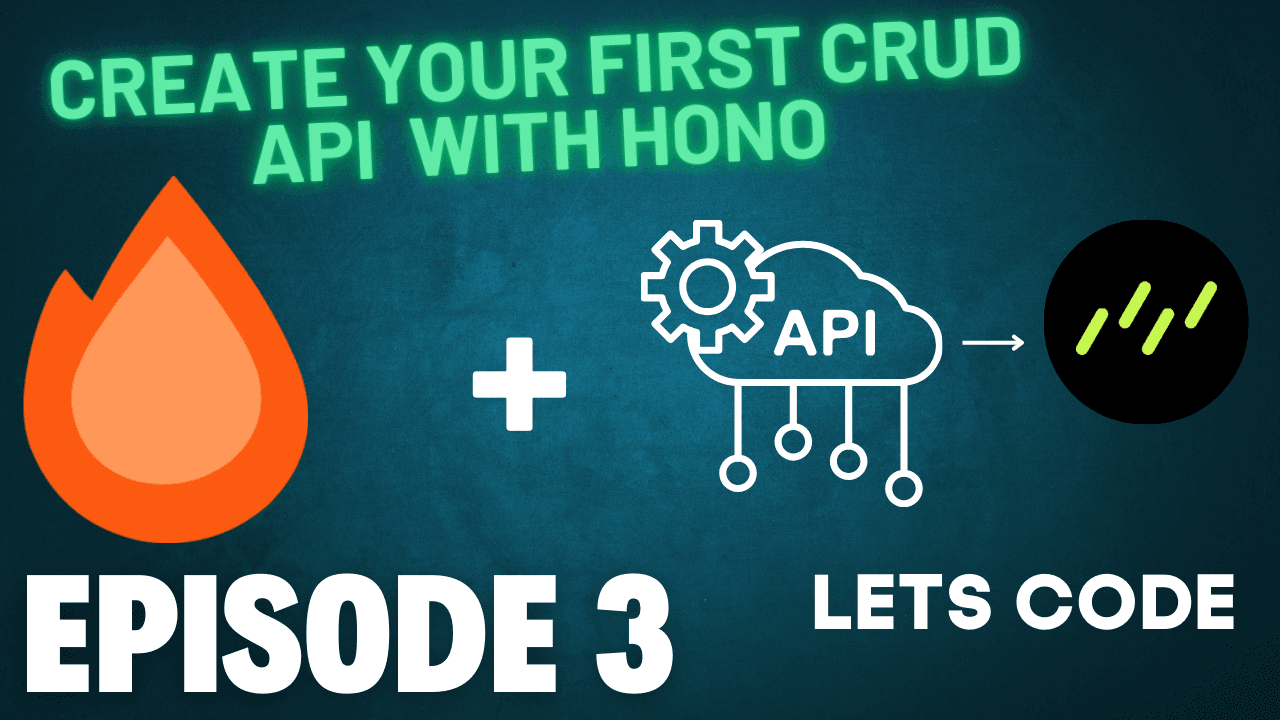
What We’ll Cover in Episode 3
In this episode, we’ll cover the essential steps to create a full CRUD API from scratch using Hono, a fast and lightweight web framework for building APIs, and SQLite, a relational database that’s simple yet powerful for small-scale projects.
Here’s what you’ll learn:
- Setting Up Hono & SQLite: We'll start by installing Hono and setting up an SQLite database. Hono is an excellent choice for building APIs due to its simplicity and performance. SQLite makes it easy to manage data without the complexity of a full-fledged database system.
- Building the CRUD Operations: The main part of the episode will be focused on creating the create, read, update, and delete operations for our API. These operations are the backbone of most web applications, and mastering them will help you in building more complex systems.
- Project Structure and Best Practices: I'll walk you through a clean and efficient project structure to keep things organized. Best practices will be highlighted, including how to structure your API routes and handle database operations in a way that scales well.
The GitHub Repo for Episode 3
You can find the complete code for this episode on the following GitHub repository:
GitHub Repo - First CRUD API with Hono
Feel free to clone the repository and follow along with the tutorial. I’ve made sure to include clear and concise comments in the code to help you understand each step.
Step-by-Step Breakdown
1. Fork or Clone the Template Repo
To get started quickly, instead of installing Hono from scratch, you can fork or clone my template repository, which serves as a base for Hono applications. This template includes the essential setup for Hono, giving you a head start on your API project.
Clone the template repo here:
Getting Started with Hono - Template Repo
Once you’ve cloned or forked the repo, navigate into your project directory and install the necessary libraries to work with SQLite.
2. Install SQLite Libraries
To interact with SQLite and add database support, you’ll need to install the following libraries:
bashCopy codenpm install drizzle-kit drizzle-orm better-sqlite3 dotenv
npm install --save-dev @types/better-sqlite3
- drizzle-kit and drizzle-orm provide an easy-to-use ORM for managing your SQLite database with Hono.
- better-sqlite3 is a performant SQLite3 library for Node.js.
- dotenv is used to manage environment variables securely.
- @types/better-sqlite3 provides TypeScript types for better-sqlite3, allowing you to get full TypeScript support.
3. Set Up the Database Structure
Now that you have the required libraries installed, let's set up the database and schema.
Step 1: Create a db
folder in your src/
directory.
Inside this folder, create the following files:
- db.sqlite: This will be your SQLite database file.
- index.ts: This file will contain the connection logic to set up your SQLite database.
- schema.ts: This will define the schema for your tables.
Here’s how to set them up:
- index.ts
typescriptCopy codeimport { drizzle } from 'drizzle-orm/better-sqlite3';
import Database from 'better-sqlite3';
// Connect to SQLite database (db.sqlite will be created in the same directory)
const sqlite = new Database('db.sqlite', { verbose: console.log });
export const db = drizzle(sqlite);
- schema.ts
typescriptCopy codeimport { sqliteTable, text, integer } from 'drizzle-orm/sqlite-core';
// Define the schema for the 'tasks' tableexport const tasks = sqliteTable('tasks', {
id: integer('id').primaryKey({ autoIncrement: true }),
title: text('title').notNull(),
description: text('description'),
status: text('status').notNull().default('pending'),
});
Step 2: Create the drizzle.config.ts
file in the root directory. This configuration will tell drizzle-kit
how to generate and migrate the database schema.
- drizzle.config.ts
typescriptCopy codeimport { defineConfig } from 'drizzle-kit';
export default defineConfig({
out: './drizzle', // Directory to output migration files
schema: './src/db/schema.ts', // Path to your schema file
dialect: 'sqlite', // Dialect is SQLite
dbCredentials: {
url: process.env.DATABASE as string, // Use the DATABASE environment variable for DB URL
}
});
Step 3: Create the .env
file in the root directory to define your database URL. This will be used in the drizzle.config.ts
.
- .env
envCopy codeDATABASE=./src/db/db.sqlite
Step 4: Create an empty drizzle
folder in the root directory. This folder will store all the migration files created by drizzle-kit
.
Step 5: Update your package.json
file with the following scripts to run migrations and generate the schema:
jsonCopy code{
"scripts": {
"db:generate": "drizzle-kit generate", // Generate migration files
"db:migrate": "drizzle-kit migrate" // Apply migrations to the database
}
}
4. Run Migrations
After setting everything up, it's time to run the migrations and generate the initial schema for the database:
- Generate migrations:
bashCopy codenpm run db:generate
- Apply migrations to the database:
bashCopy codenpm run db:migrate
This will create the tasks
table in your db.sqlite
file.
5. Write the Endpoint Logic
Now that the database and schema are set up, it's time to interact with it in your API. Below is the complete working logic for handling CRUD operations using Hono and Drizzle ORM.
Here's how you can define your endpoints to interact with the tasks
table in the SQLite database:
typescriptCopy codeimport { Hono } from 'hono';
import { db } from './db';
import { tasks } from './db/schema';
import { eq } from 'drizzle-orm';
const app = new Hono();
// Create Task
app.post('/tasks', async (c) => {
const { title, description } = await c.req.json();
const newTask = await db.insert(tasks).values({
title,
description,
}).returning();
return c.json(newTask);
});
// Get All Tasks
app.get('/tasks', async (c) => {
const allTasks = await db.select().from(tasks);
return c.json(allTasks);
});
// Get Task by ID
app.get('/tasks/:id', async (c) => {
const taskId = c.req.param('id');
const task = await db.select().from(tasks).where(eq(tasks.id, Number(taskId)));
return c.json(task);
});
// Update Task
app.put('/tasks/:id', async (c) => {
const taskId = c.req.param('id');
const { title, description, status } = await c.req.json();
const updatedTask = await db.update(tasks).set({
title,
description,
status,
}).where(eq(tasks.id, Number(taskId))).returning();
return c.json(updatedTask);
});
// Delete Task
app.delete('/tasks/:id', async (c) => {
const taskId = c.req.param('id');
await db.delete(tasks).where(eq(tasks.id, Number(taskId)));
return c.json({ message: 'Task deleted successfully' });
});
export default app;
Explanation of the Endpoints:
- POST
/tasks
: Creates a new task in thetasks
table. The request body must includetitle
anddescription
. - GET
/tasks
: Fetches all tasks from thetasks
table. - GET
/tasks/:id
: Fetches a single task by ID from thetasks
table. Theid
is passed as a URL parameter. - PUT
/tasks/:id
: Updates a task by ID. The request body must include thetitle
,description
, andstatus
fields. - DELETE
/tasks/:id
: Deletes a task by ID from thetasks
table.
Final Notes:
- All routes use the Drizzle ORM to interact with the SQLite database (
db.sqlite
). - You can extend these CRUD operations to add more functionality as needed as we will do in the upcoming videos