Enrise Tech - Your coding community 😉🚀|| Operator🐍 and Condition🐍 💻
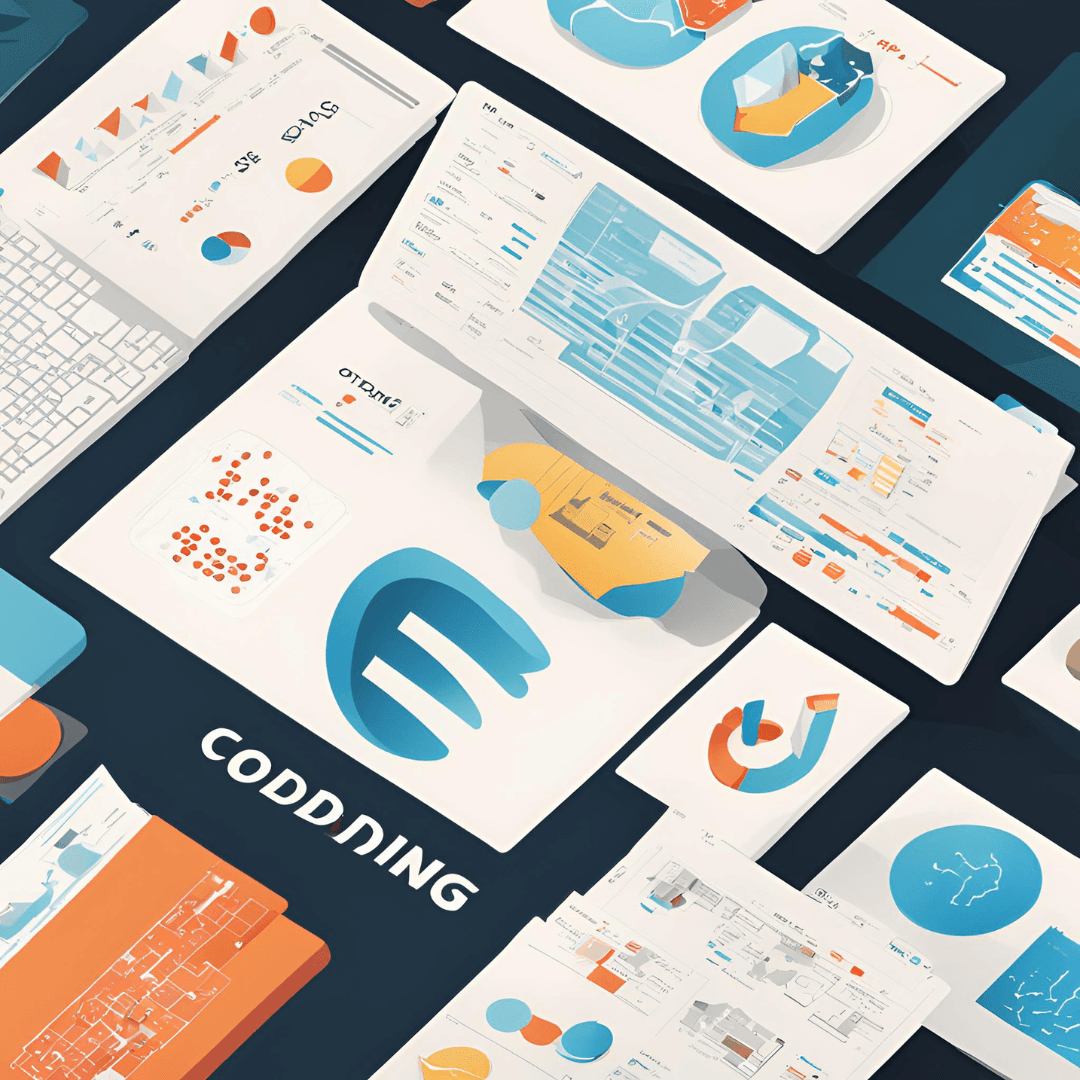
Operators and Conditions: Outline
- Introduction to Operators
- Overview of operators in programming
- Types of operators: arithmetic, comparison, logical, assignment, etc.
- Arithmetic Operators
- Addition, subtraction, multiplication, division
- Modulus and exponentiation operators
- Comparison Operators
- Equal to, not equal to
- Greater than, less than
- Greater than or equal to, less than or equal to
- Logical Operators
- AND, OR, NOT operators
- Assignment Operators
- Simple assignment vs. compound assignment
- Examples and use cases
- Conditional Statements
- Introduction to if, else if (elif), and else statements
- Syntax and basic examples
- Code Examples !
1. Introduction to Operators
- Operators in programming are symbols that perform operations on variables and values. They include arithmetic (like + and -), comparison (like == and >), logical (like && this is AND and || this is OR), and assignment (like = and +=) operators.
- Example:
+
in2 + 3
performs addition, resulting in5
.
2. Arithmetic Operators
- Arithmetic operators perform mathematical calculations on numerical values. They include addition (
+
), subtraction (), multiplication (
), division (/
), modulus (%
), and exponentiation (*
). - Example:
10 % 3
returns the remainder when 10 is divided by 3, which is1
3. Comparison Operators
- Comparison operators compare two values and return a boolean result (
true
orfalse
). They include equal to (==
), not equal to (!=
), greater than (>
), less than (<
), greater than or equal to (>=
), and less than or equal to (<=
). - Example:
5 > 3
returnstrue
because 5 is greater than 3.
4. Logical Operators
- Logical operators are used to combine conditional statements. They include AND (
&&
), OR (||
), and NOT (!
) operators. - Example:
(x > 0 && x < 10)
checks ifx
is greater than 0 and less than 10.
5. Assignment Operators
- Assignment operators are used to assign values to variables. They include simple assignment (
=
) and compound assignment operators (+=
,-=
,*=
,/=
, etc.). - Example:
x += 5
is equivalent tox = x + 5
, incrementingx
by 5.
6: Conditional Statements
- Conditional statements allow us to make decisions in code based on specified conditions. They include
if
,else if
(often abbreviated aselif
in Python Specifically), andelse
statements. - Example if (isNightTime ) then sunGoesDown
7. Code Examples
Now lets continue working in our main.py file in our replr setup.
Write the following code in your replr window.
# Operators in action ( this is called a comment FYI: its ignored and not read by the computer, but this is for the human or user think of it as a guide in the code )
# Lets all the code we wrote in the previous lesson
# Operation and Conditions code only below
number_a = 10
number_b = 5
sum_result = number_a + number_b # Addition
print(sum_result) # Output: 15
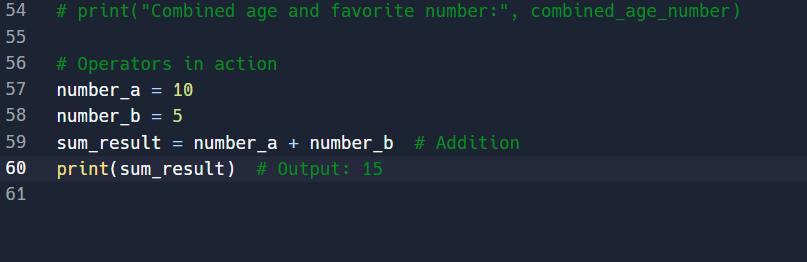
Arithmetic Operations
Now we will add Arithmetic operations, see the code below, you need to type.
This will show an example of each of the Arithmetic operators we can do.
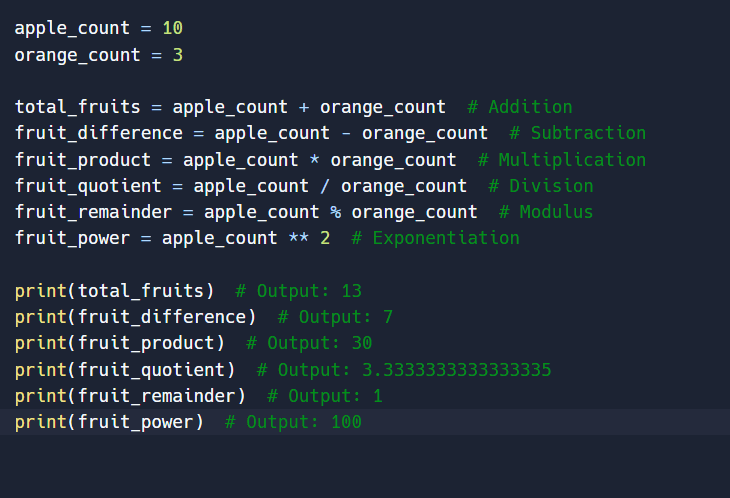
Comparison Operators
Now we will look at comparing 2 or more variables to each other to get either a true or false value.
Copy this code into your editor:
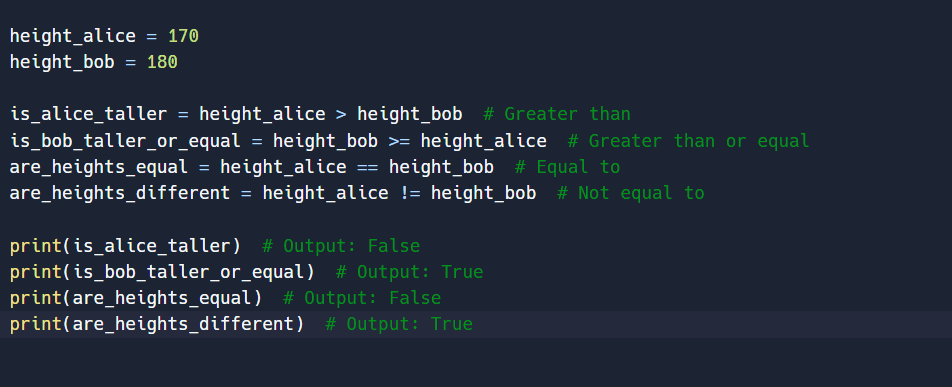
Logical Operators
These add some logic to our code and bring some life into it, because it needs to make decisions based on this logic.
Copy the code below and paste into your code editor.
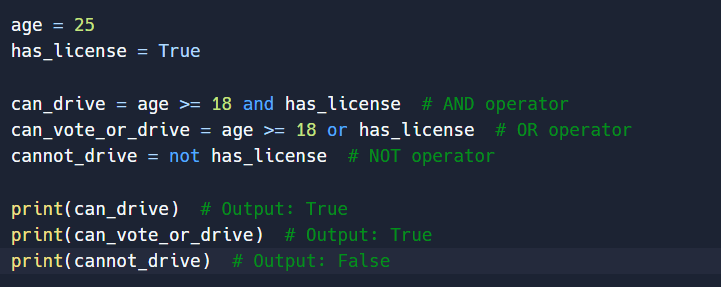
Assignment Operators
These allow us to update our variables or values in our code.
See the example below and ensure you write this code on your editor.
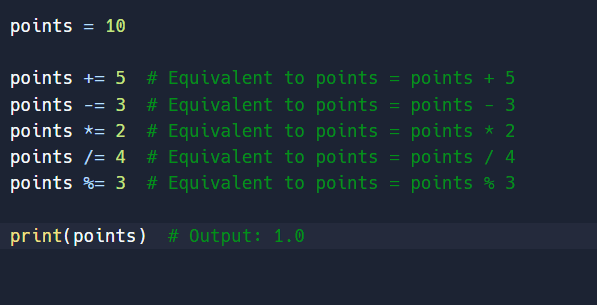
Conditional Statements
These work hand in hand with the logical operations, in that they bring life to your code too.
Lets see how we write code that tells us its hot or not, based on the weather.
See example and type this put and run it on your side
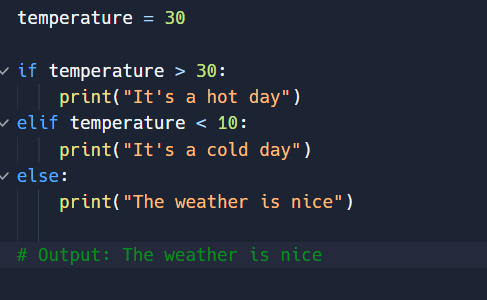
The examples above looked at provide a clear and concise guide to someone that either has not or has limited coding knowledge. Join us in the next lesson as we build on top of this