Enrise Tech - Your coding community ๐๐๐ Introduction to Python Basics ๐
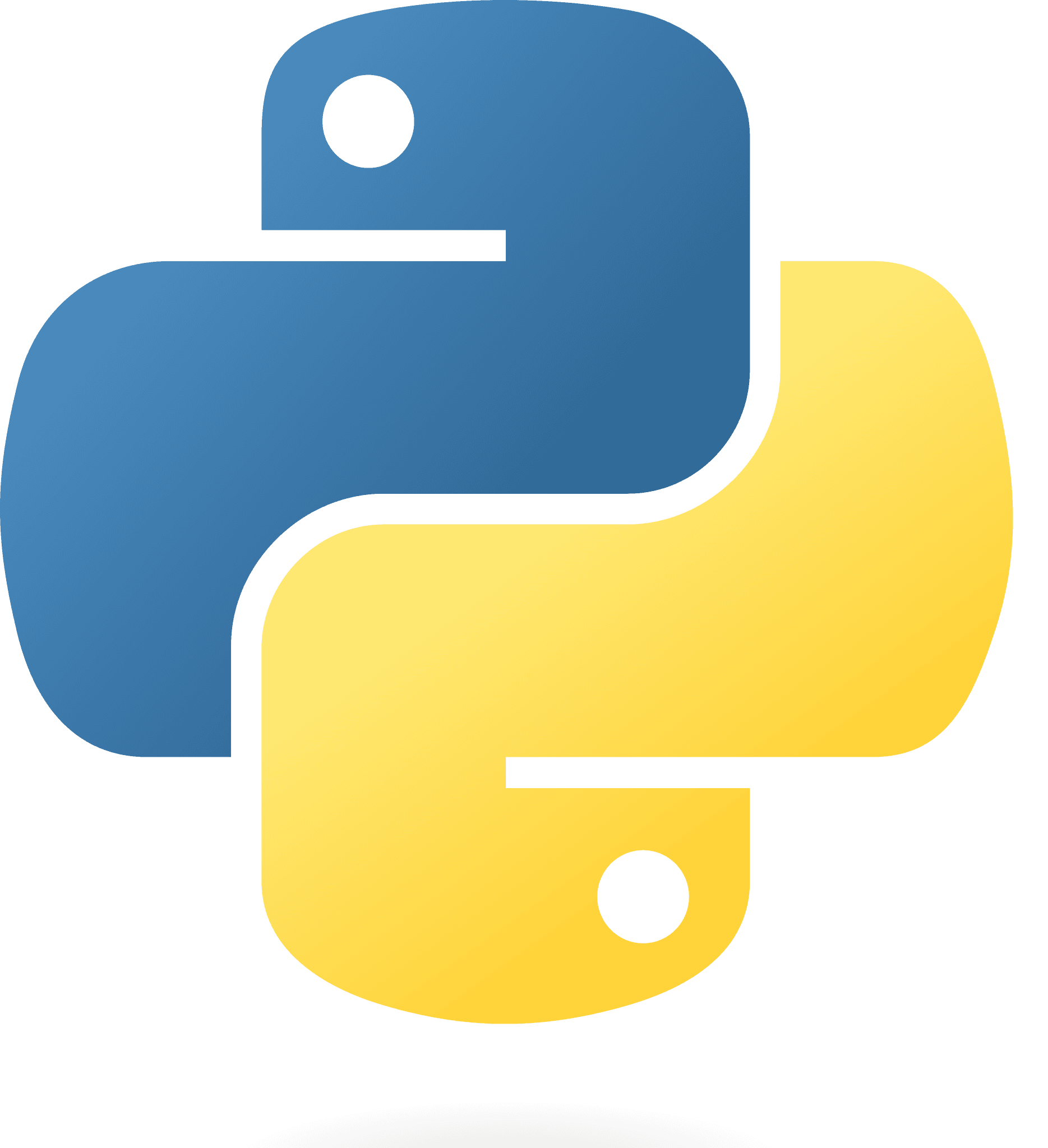
Outline
Introduction to Python Basics
- Variables and Data types
- Basic operations
Tutorial: Understanding Variables and Data Types in Python
Welcome to the next step in your Python journey! In this tutorial, you'll learn about variables and data types. Let's dive in and start coding in main.py
using Replit.
Step 1: Open Previous lesson Replit
- Visit Replit.
- Use the previous replr we coded along too.
- Youโll see a file named
main.py
โthis is where youโll write your code.
Step 2: Lets understand variables
Variables are containers for storing data values. Let's create some variables:
I like to think of code as telling a story, lets tell a story about Alice using what we know so far.
Zodwa is a 30 year old female, that is 5.7 meters tall ( Damn, pretty tall ) and is a student.
Before we start, make sure you open the replr and are inside the main.py file.
Remove the 'Hello World' print statement and write the follpwing below
# Creating variables
name = "Zodwa"
age = 30
height = 5.7
is_student = True
# Printing variables
print(name)
print(age)
print(height)
print(is_student)
This is how your code will look now on your replr. See screenshot below
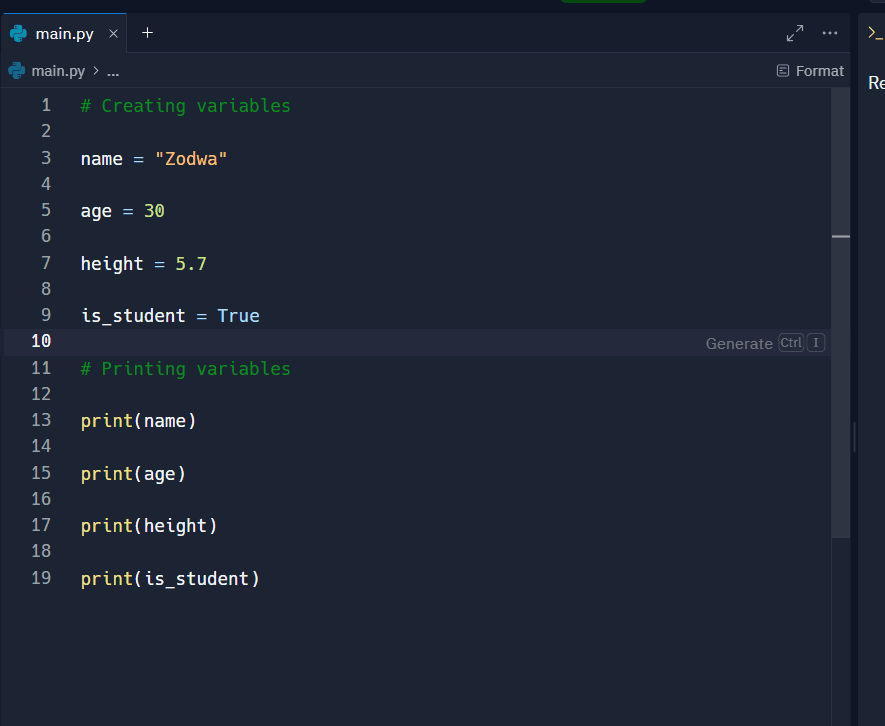
Explanation
We have used Python to tell our story using variables to store information about Zodwa. The Print will just ( you guessed it ) print information to the screen.
Output
Click run to see the output of the code, see output below:
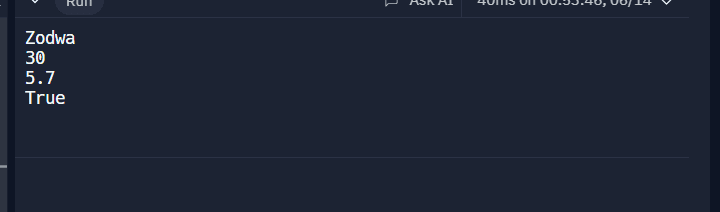
Step 3: What are Data types?
Python has various data types, Remember these describe our variables, what can you remember about Zodwa ?
age -> the Datatype is known as Integer ( whole numbers eg 30 )
height -> the Datatype is known as Floats ( In Maths we call it decimal eg 5.7 )
name -> the Datatype is known as String ( Text eg 'Zodwa')
is_student -> the Datatype is known as Boolean ( True or False eg: True)
To make sure we accurate lets show you in Python how to view the datatypes of your variables: Write the following code below and run it to see the output
# Data types examples
print(type(name)) # Output: <class 'str'>
print(type(age)) # Output: <class 'int'>
print(type(height)) # Output: <class 'float'>
print(type(is_student)) # Output: <class 'bool'>
Run the code and check see the output below to ensure its consistent with yours:
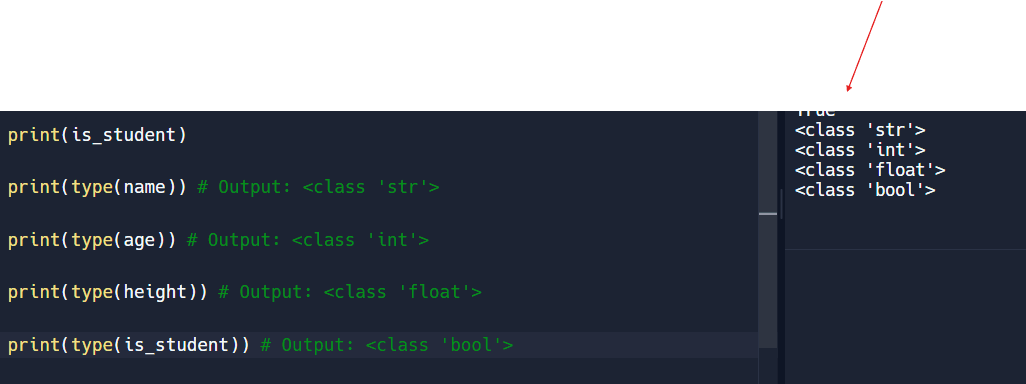
Step 4: Basic Operations with Variables
You can perform operations with variables based on their data types.
Meaning we can add, minus, subtract and divide just like you learnt in Mathematics, with variables but based on their datatypes.
Type the following in your REPLR and send us your replr links.
# Arithmetic operations
sum_age_height = age + height
print("Sum of age and height:", sum_age_height)
# String concatenation full_message = "Hello, my name is " + name print(full_message) # Boolean operations is_adult = age > 18print("Is an adult:", is_adult)
See screenshot of the above piece of code.
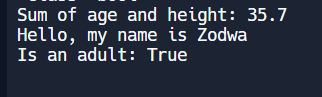
To write to us go to our instagram link
Step 5: Hands on Exercise
Now, it's your turn! Write the following inside your main.py file:
1) Declares variables for your name, age, favorite number, and whether you're a student.
2) Prints the type of each variable.
3) Performs an operation using these variables and prints the result
I will only write the first part of the challenge, the rest I will leave it to you to figure out. See screenshot of my code below
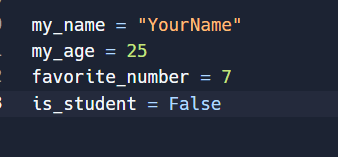
To see complete Code lesson, please see link below to REPLR.